Attributes
Attributes can be assigned to fields in model classes to modify their behavior on both the front end and the back end.
UIName
The UIName
attribute sets the name of the record when searching for records in a select box from the UI.
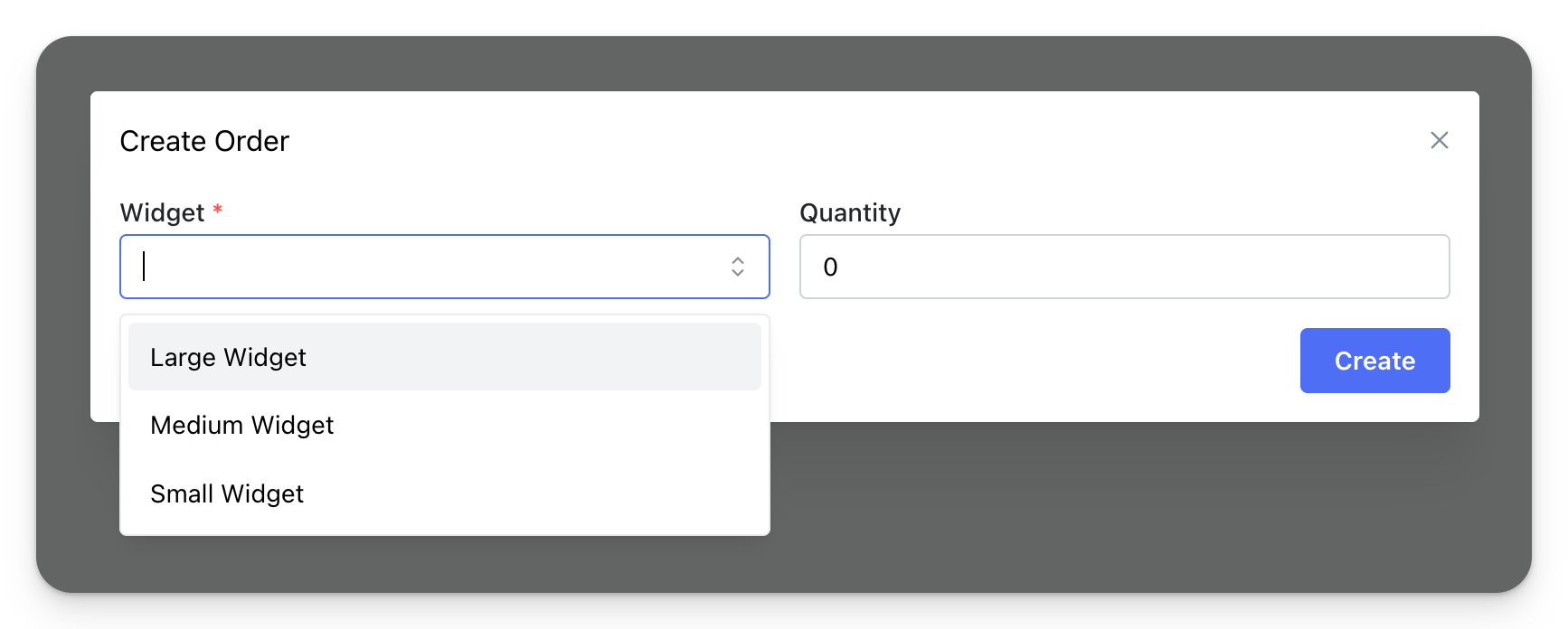
Project.Common / Entities / Widget.cs
UIDescription
The UIDescription
attribute sets the description of the record when searching for records in a select box from the UI.
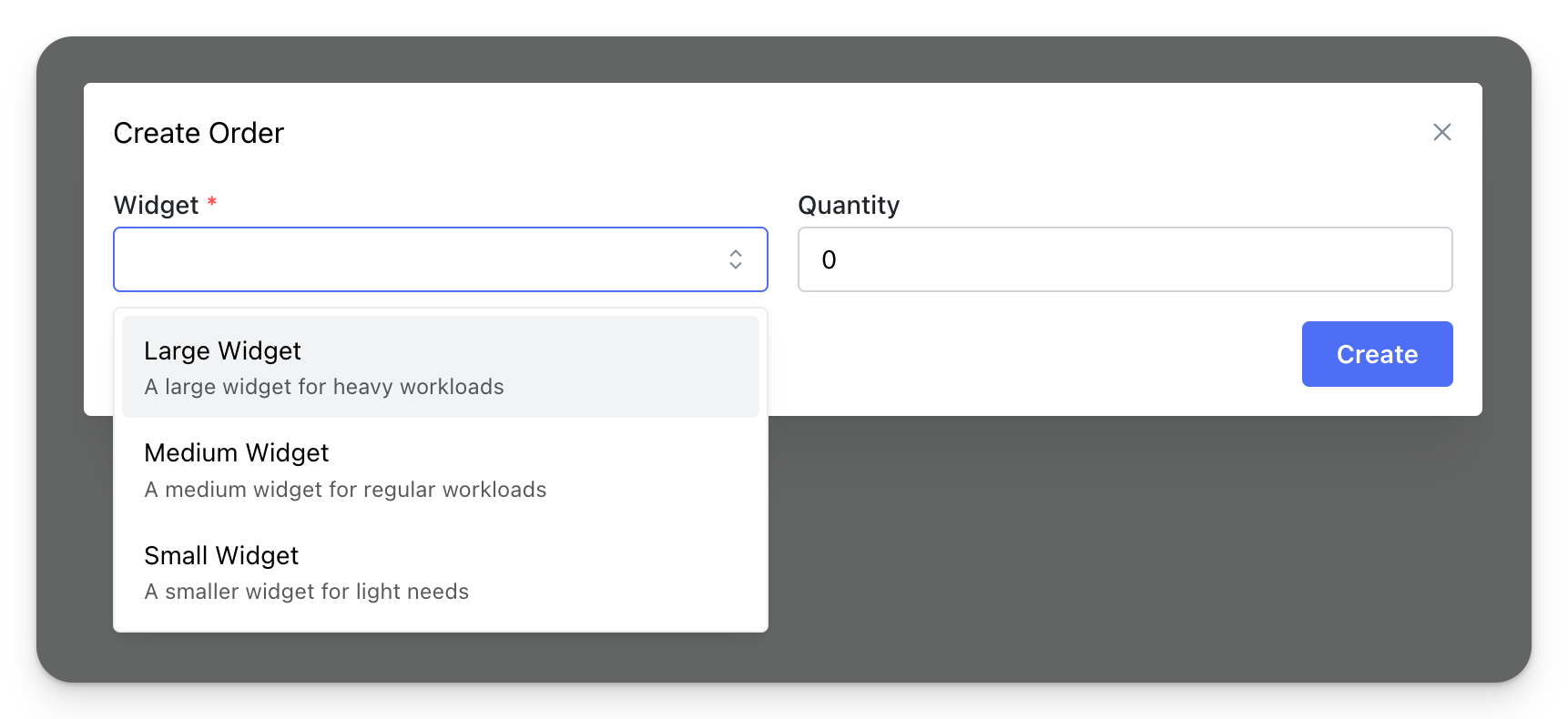
Project.Common / Entities / Widget.cs
UIDisplayName
The UIDisplayName
attribute sets the label for the field on forms in the user interface (UI).
Project.Common / Entities / Widget.cs
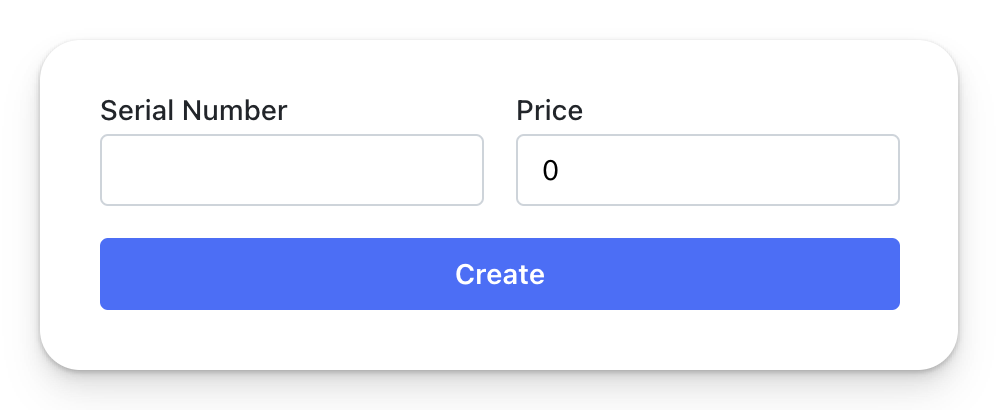
UIRequired
The UIRequired
attribute ensures that a value is provided both on the UI and the backend. An asterisk will appear on the form, along with form validation. If the value is not provided and sent to the server, the server will return a 400 error.
Project.Common / Entities / Widget.cs
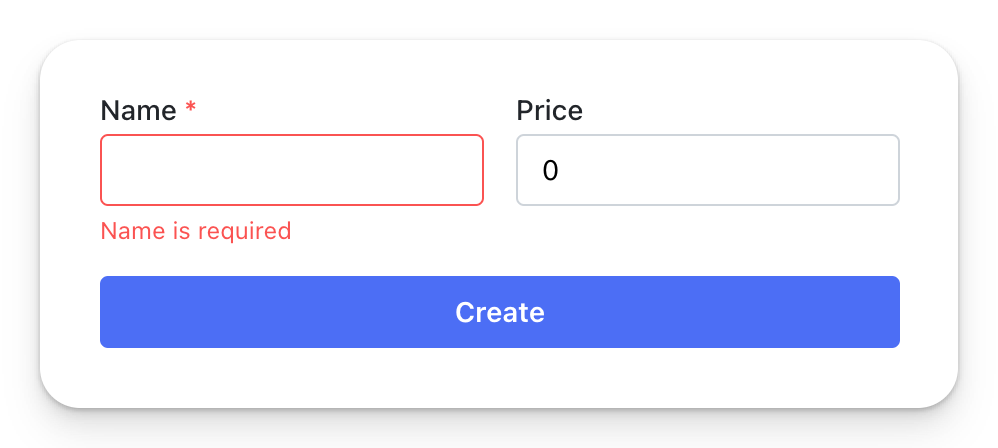
UIRecommended
The UIRecommended
attribute displays a blue cross, indicating to the user that filling out the field is recommended.
Project.Common / Entities / Widget.cs
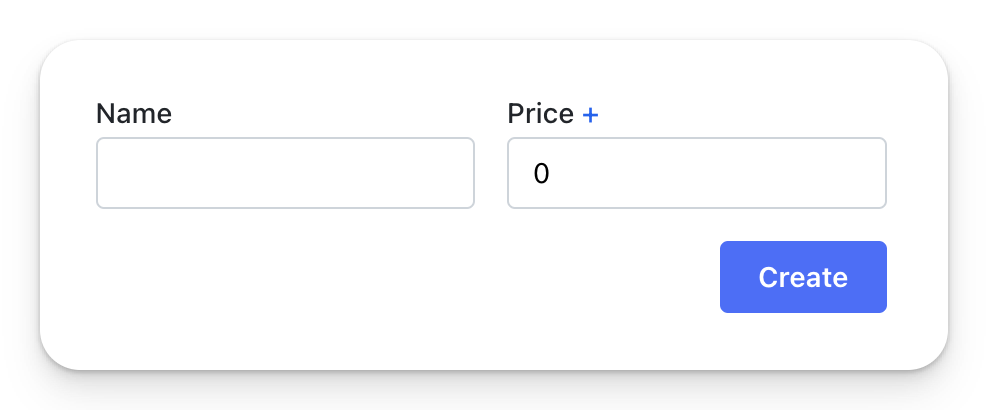
UIHide
The UIHide
attribute ensures that a field is accessible only on the server within the service layer of your application. This field cannot be displayed in the user interface or queried from JavaScript.
Project.Common / Entities / Widget.cs
The field can be made queryable by passing a value of true into the first parameter.
Project.Common / Entities / Widget.cs
UIReadOnly
The UIReadOnly
attribute ensures that a field can only be read from the UI, even if the user has Update or Create permissions. The only way to update read-only fields is by using the DbContext directly to bypass the Service Logic pipeline.
Project.Common / Entities / Widget.cs
UIOption
The UIOption
attribute allows the selection of an option record.
Options are created from the Admin Dashboard.
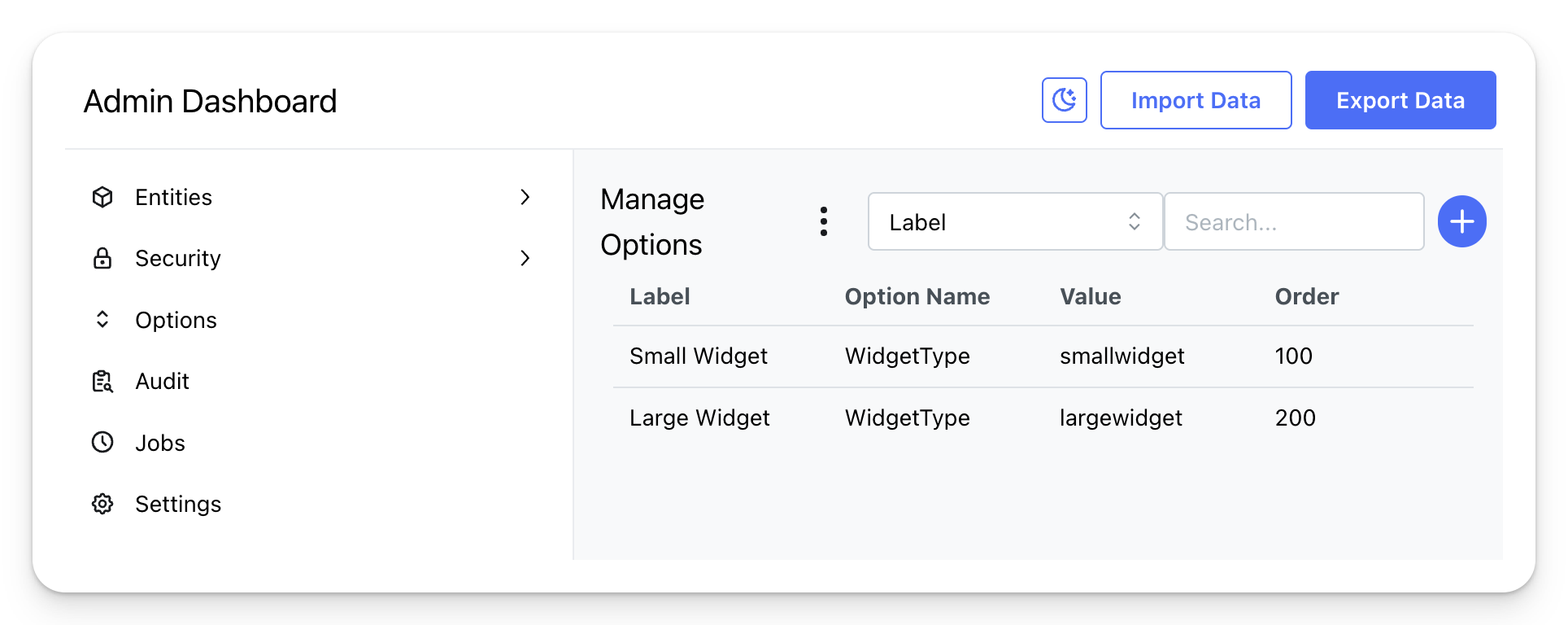
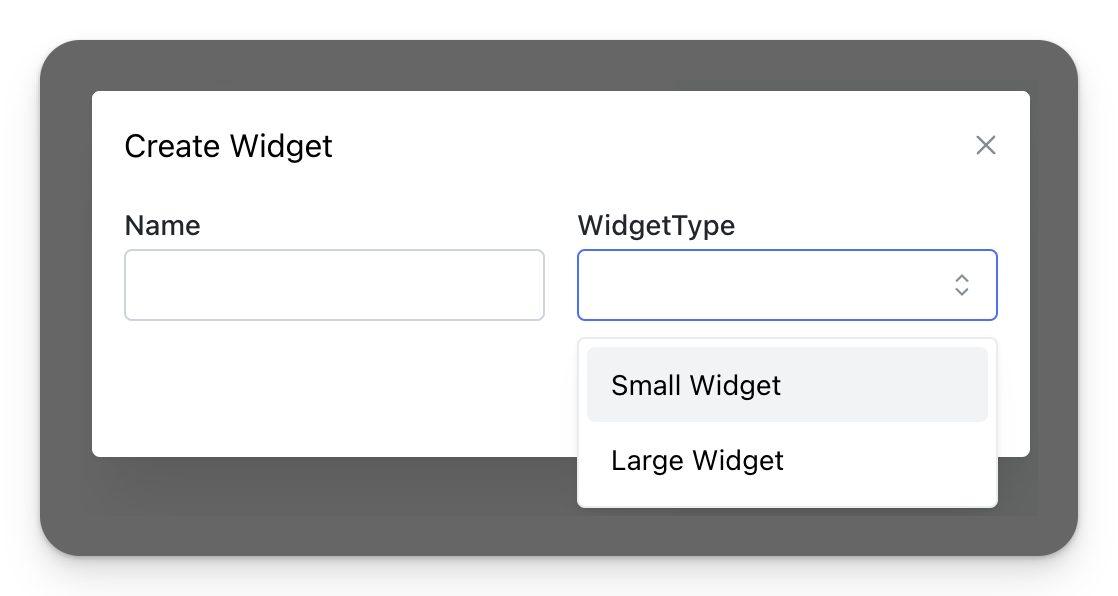
Project.Common / Entities / Widget.cs
UIDateFormat
The UIDateFormat
attribute defines the display format for DateTime fields.
Set this parameter to any valid Day.js format.