Quickstart
This guide will get you up and running with Xams and covers the following:
- Creating a Xams Api project
- Creating a Xams NextJS \ React project
- Creating tables
- Displaying and creating records from a datatable
- Writing service logic
- Creating forms
Prerequisites
Install EF Core Tools
Ensure you have version 8+ of the Entity Framework Core tools installed.
Terminal
Install Xams CLI
Install the Xams CLI if you haven't installed it yet.
Terminal
Create Api Project
- Create the directory you want your project to live in.
- Open the command prompt or terminal in the directory.
- Execute the Xams CLI. Choose
Create Api Project
.
Terminal
- Enter the name of your project. All of the c# project will be prefixed with this name.
- Open the solution in your IDE of choice. The projects contained in this solution are:
- Project.Common: Classes shared across projects including Entities
- Project.Data: Entity Framework migrations as well as the DbContext
- Project.Services: All service layer logic
- Project.Web: The api endpoints
- The project is configured to use SQLite by default. Run the following commands in the Project.Data folder to create the first migration and update the database.
Terminal - / Project.Data
- Run the project using HTTPS. The project will launch the
Admin Dashboard
automatically. It may take a moment to start.
Create NextJS Project
- Open the command prompt or terminal in the directory to create the React \ NextJS project.
- Execute the Xams CLI. Choose
Create React \ NextJS Project
.
Terminal
- Enter the name of your web app. A folder with the name of your web app will be created and includes all of the web app files.
- Open the folder in your code editor of choice.
- In the directory of the web app execute the following code.
Terminal
- With the Api running, open the NextJS project in the browser (http://localhost:3000?userid=f8a43b04-4752-4fda-a89f-62bebcd8240c)
A new Xams project isn't configured with Authentication or Authorization but still has users. Whenever the api starts, it creates system records. One of those system records is the "SYSTEM" user. The Id of this user is always f8a43b04-4752-4fda-a89f-62bebcd8240c.
Create an Entity
- In the "Common" project create a new class file in the Entities folder and name it "Widget.cs"
- Paste the below code in the file.
Project.Common / Entities / Widget.cs
- Add the Widget class to the DataContext class.
Project.Data / DataContext.cs
- In the Project.Data directory execute the code below to create a migration and update the database.
Terminal
- Start the project
Create a Datatable
- Paste the following code in index.tsx
src / pages / index.tsx
This will create the following table.
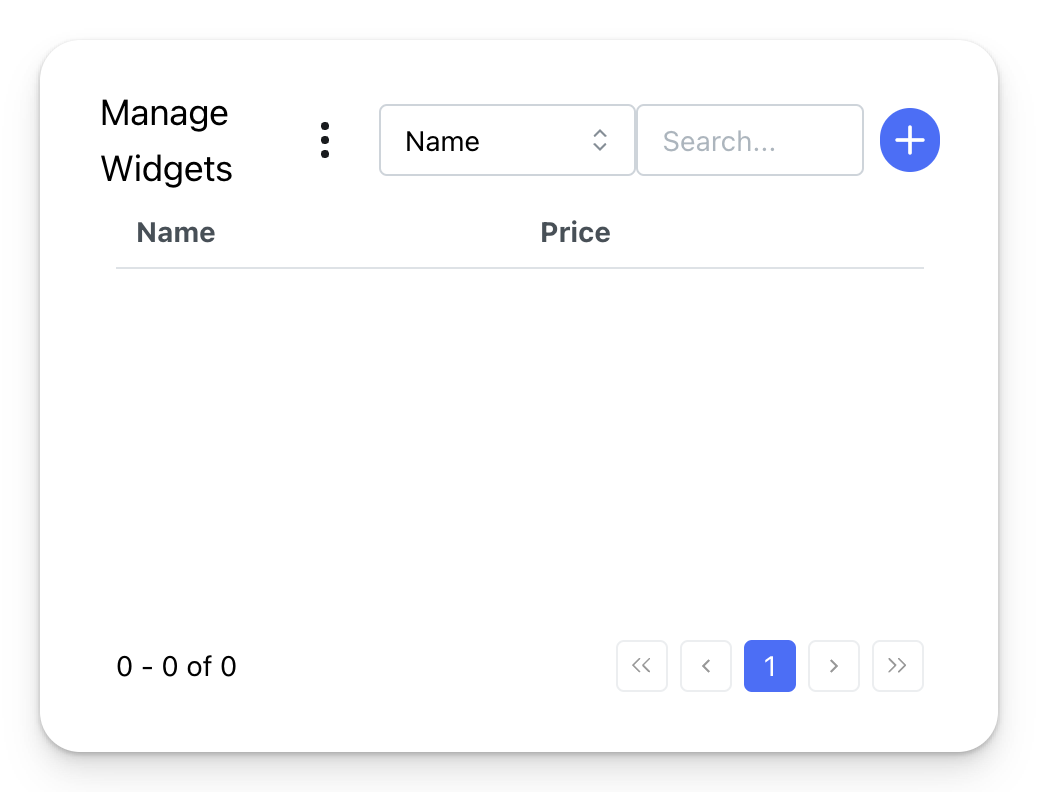
If you click the "Add" button on the table, a form will open where a new record can be created.
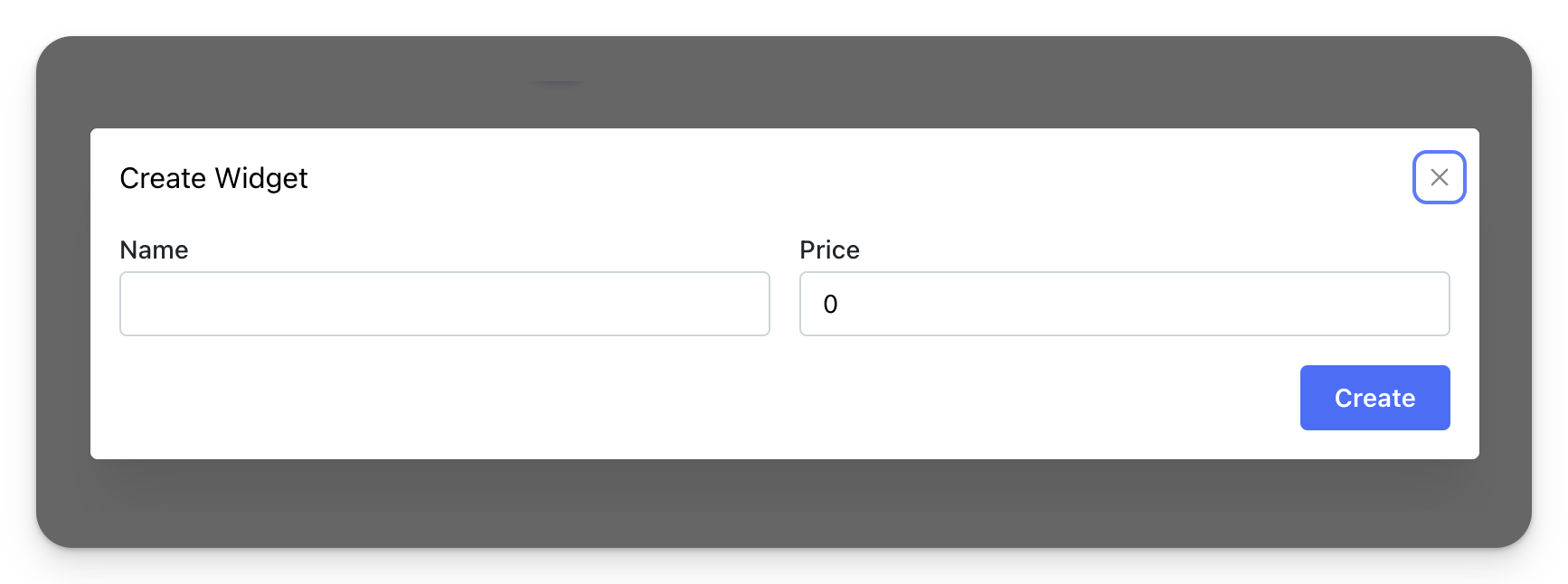
Add Service Logic
We can have the service layer automatically set a price for the Widget based on the number of Widgets in the database.
- Create a new class file in the Project.Services/ServiceLogic folder. Name it "WidgetService.cs"
- Replace the class in the file with the code below.
Project.Services / ServiceLogic / WidgetService.cs
Create a Record
- Replace the code in index.tsx with the following.
src / pages / index.tsx
When the button is clicked, a Widget record will be created with the provided data.
Create a Form
- Replace the code in index.tsx with the following.
src / pages / index.tsx
This will create the below form:
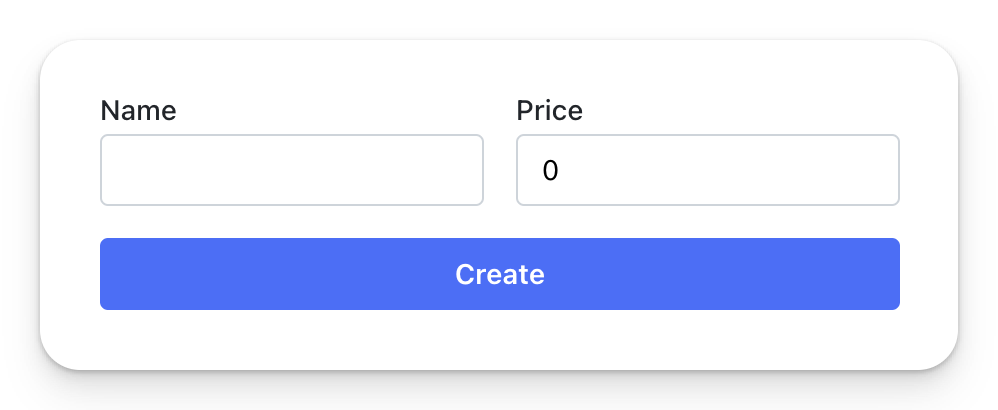