Security
Security is configured in the Admin Dashboard by using Teams, Roles, and Permissions.
Schema
The diagram below shows the relationships between the security entities. Users are assigned to Teams, while Roles can be assigned to both Teams and Users. Permissions are assigned to Roles. Users inherit permissions from their Roles and Team's Roles.
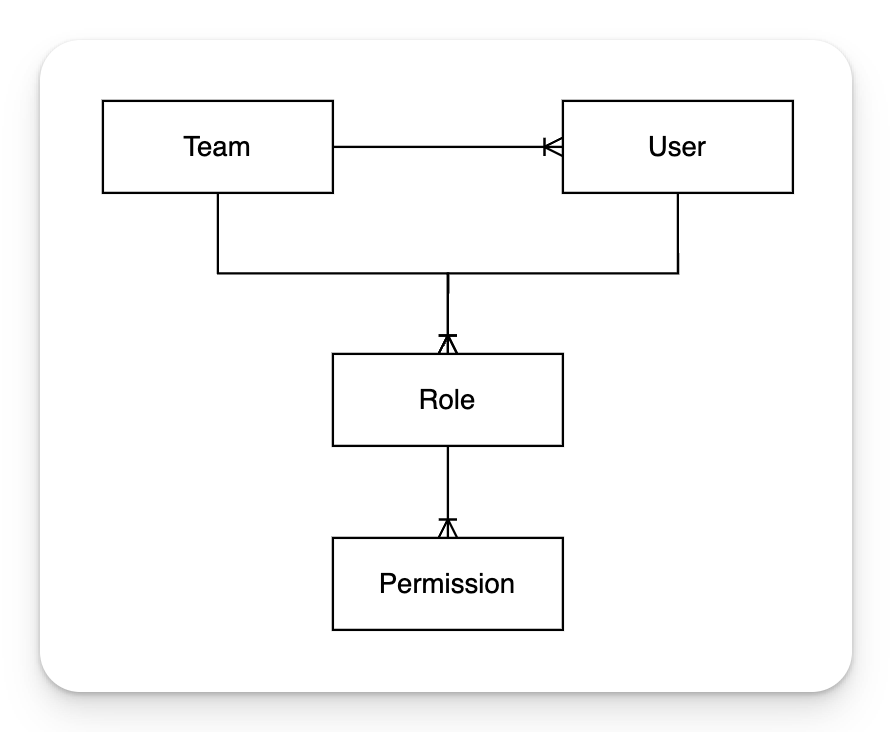
Permissions
Permissions for Roles can be configured from the Admin Dashboard under Security -> Roles. Permissions are additive, meaning they accumulate from multiple roles.
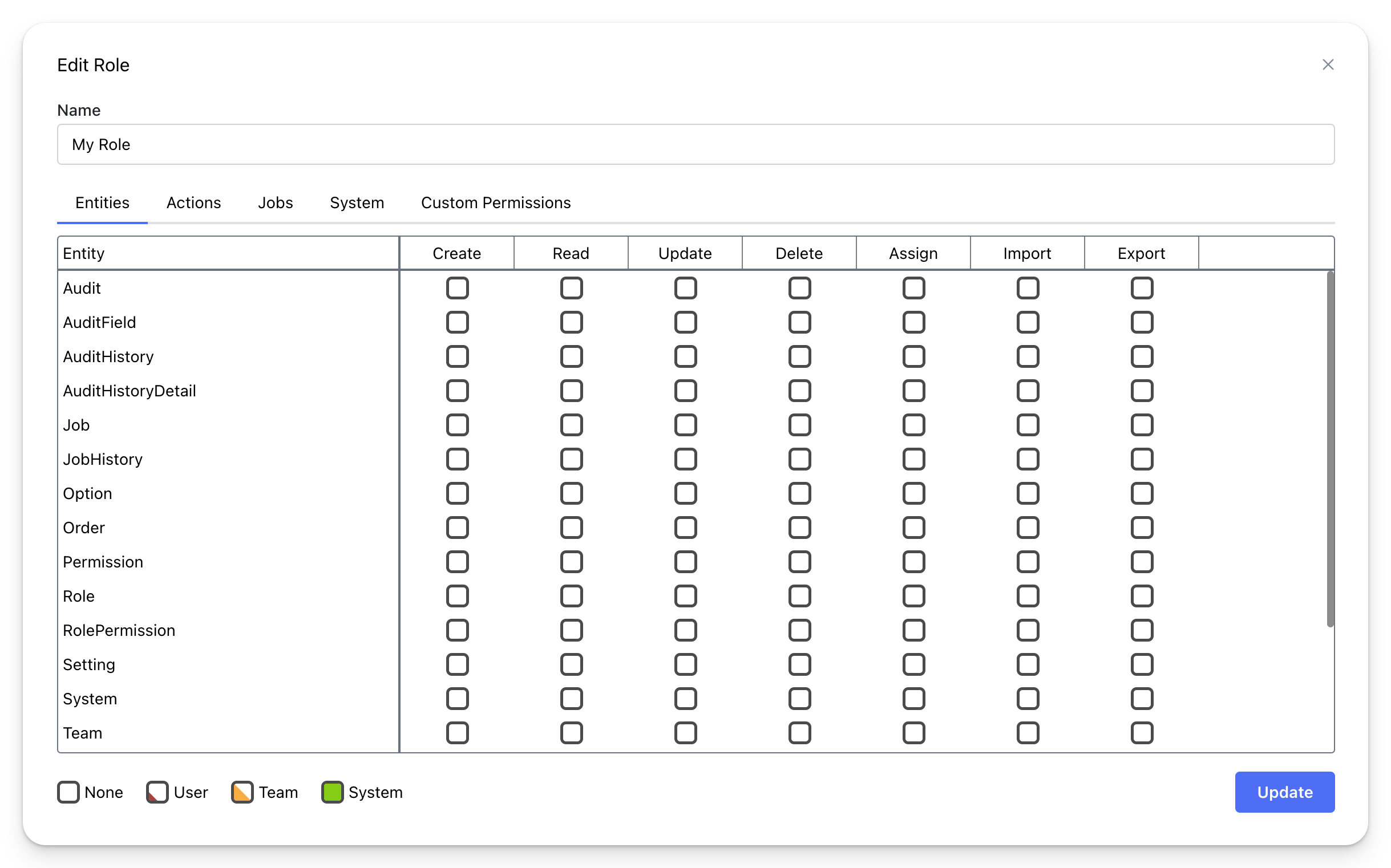
Create, Read, Update, Delete, and Assign permissions can be set at the User
, Team
, or System
level. Entities that inherit from the BaseEntity
class will have OwningUserId
and OwningTeamId
fields, which determine the record owner.
The OwningUserId
and OwningTeamId
fields can be set simultaneously, but at least one must always be specified.
- User-level permissions: Users can only create, read, update, or delete records
where the
OwningUserId
field is set to their user ID. - Team-level permissions: Users can create, read, update, or delete records
where the
OwningUserId
field is set to their user ID or where theOwningTeamId
field is set to a team they belong to. - System-level permissions: Users can create, read, update, or delete any record in the system.
If a table does not inherit from the BaseEntity
class, System-level permissions are required to Create, Read, Update, and Delete records. In this case, records cannot be assigned or have ownership, as they lack the OwningUserId
and OwningTeamId
fields.
Assign
-
User-level assign permissions: When creating records, users can only assign the record to themselves (
OwningUserId
). If this field is not set, it defaults to the user creating the record. -
Team-level assign permissions: Users can assign the
OwningUserId
to themselves or any user in the teams they belong to. Additionally, they can set theOwningTeamId
to any of the teams they are a member of. -
System-level assign permissions: Users can assign the record to any user (
OwningUserId
) or team (OwningTeamId
) in the system.
Import & Export
To enable the ability to export, import, and download the Import Template, the following permissions must be enabled: ACTION_TABLE_ExportData
, ACTION_TABLE_ImportData
, and ACTION_TABLE_ImportTemplate
. These permissions are located under Role -> System. Once these are enabled, Import and Export permissions can be configured for each table individually.
-
Import permissions: Users with import permissions can import data using the provided Excel Export Template.
-
Export permissions: Users with export permissions can download the current table view as an Excel file.
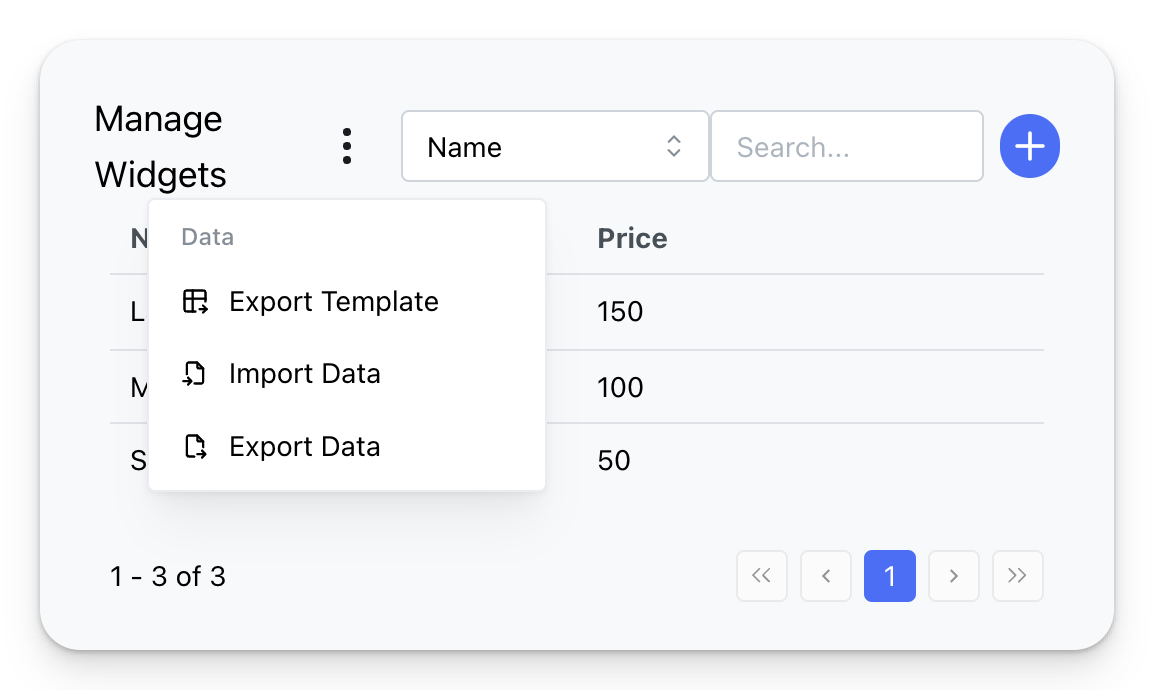
Custom Permissions
Custom permissions are useful for creating permissions that are tailored to the specific needs of your application, allowing for detailed control over access to custom functionality.
Custom permissions can be created from the Admin Dashboard by navigating to Security -> Permissions. Once created, custom permissions can be assigned to a role by selecting the Custom Permissions tab when updating the role.
Checking Permissions
In React, you can check if a user has any or all permissions from an array by using the hasAnyPermissions
or hasAllPermissions
methods from the useAuthRequest
hook.
src / components / MyComponent.tsx
From the ServiceLogic use the Permissions method of the ServiceContext to return an array of matching permissions the user has.
Project.Services / Logic / MyEntityService.cs
Permission Names
Table permissions follow the convention TABLE_(TableName)_(Operation)_(Level)
.
For example, the System-level read access permission for the Widget entity is TABLE_Widget_READ_SYSTEM
.
The operation can be one of the following: CREATE
, READ
, UPDATE
, DELETE
, or ASSIGN
. The level can be USER
, TEAM
, or SYSTEM
.
Import and Export permissions follow the convention TABLE_(TableName)_IMPORT
and TABLE_(TableName)_EXPORT
.